A newer version of this document is available. Customers should click here to go to the newest version.
Visible to Intel only — GUID: GUID-91A0DB1B-8317-4BBE-9942-93CD39914555
Visible to Intel only — GUID: GUID-91A0DB1B-8317-4BBE-9942-93CD39914555
Use of RTL Libraries for FPGA
A static library is a single file that contains multiple functions. You can create a static library file using register transfer level (RTL). You can then include this library file and use the functions inside your SYCL* kernels.
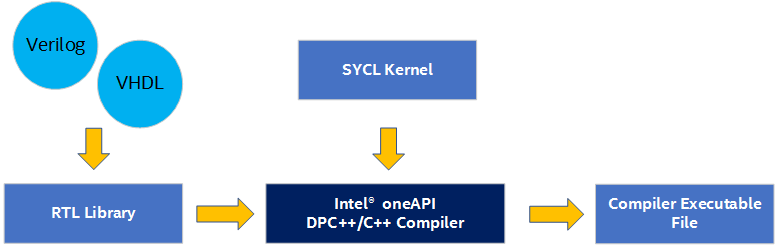
To generate libraries that you can use with SYCL, you need to create the following files:
File or Component |
Description |
---|---|
RTL modules |
|
RTL source files |
Verilog, System Verilog, or VHDL files that define the RTL component. Additional files such as Intel® Quartus® Prime IP File (.qip), Synopsys Design Constraints File (.sdc), and Tcl Script File (.tcl) are not allowed. |
eXtensible Markup Language File (.xml) |
Describes the properties of the RTL component. The Intel® oneAPI DPC++/C++ Compiler uses these properties to integrate the RTL component into the SYCL pipeline. |
Header file (.hpp) |
A header file containing valid SYCL kernel language and declares the signatures of functions implemented by the RTL component. |
Emulation model file (SYCL-based) |
Provides a C++ model for the RTL component that is used only for emulation. Full hardware compilations use the RTL source files. |
SYCL Functions |
|
SYCL source files (.cpp) |
Contains definitions of the SYCL functions. These functions are used during emulation and full hardware compilations. |
Header file (.hpp) |
A header file describing the functions to be called from SYCL in the SYCL syntax. |
The format of the library files is determined by which operating system you compile your source code on, with additional sections that carry additional library information.
On Linux* platforms, a library is a .a archive file that contains .o object files.
On Windows* platforms, a library is a .lib archive file that contains .obj object files.
You can call the functions in the library from your kernel without the need to know the hardware design or the implementation details of the underlying functions in the library. Add the library to the icpx command line when you compile your kernel.
Creating a library is a two-step process:
Each object file is created from an input source file using the fpga_crossgen command.
An object file is effectively an intermediate representation of your source code with both a CPU representation and an FPGA representation of your code.
An object can be targeted for use with only one Intel® high-level design product. If you want to target more than one high-level design product, you must generate a separate object for each target product.
Object files are combined into a library file using the fpga_libtool command. Objects created from different types of source code can be combined into a library, provided all objects target the same high-level design product.
A library is automatically assigned a toolchain version number and can be used only with the targeted high-level design product with the same version number.

Create Library Objects From Source Code
You can create a library from object files from your source code. A SYCL-based object file includes code for CPU and hardware execution of CPU capturing for use in host and emulation of the kernel.
Create an Object File From Source Code
Use the fpga_crossgen command to create library objects from your source code. An object created from your source code contains information required both for emulating the functions in the object and synthesizing the hardware for the object functions.
The fpga_crossgen command creates one object file from one input source file. The object created can be used only in libraries that target the same high-level design tool. Also, objects are versioned. Each object is assigned a compiler version number and be used only with high-level design tools with the same version number.
Create a library object using the following command:
fpga_crossgen <rtl_spec>.xml --emulation_model <emulation_model>.cpp --target sycl -o <object_file>
The following table describes the parameters:
Parameter |
Description |
---|---|
<source_file> |
An XML file name that specifies the details about your RTL library. |
--target |
Targets an Intel® high-level design tool (sycl) for the library. The objects are combined as object files into a SYCL library archive file using the fpga_libtool. |
-o |
Optional flag. This option helps you specify an object file name. If you do not specify this option, the object file name defaults to be the same name as the source code file name but with an object file suffix (.o or .obj). |
Example command:
fpga_crossgen lib_rtl_spec.xml --emulation_model lib_rtl_model.cpp --source sycl --target sycl -o lib_rtl.o
Packaging Object Files into a Library File
Gather the object files into a library file so that others can incorporate the library into their projects and call the functions that are contained in the objects in the library. To package object files into a library, use the fpga_libtool command.
Before you package object files into a library, ensure that you have the path information for all of the object files that you want to include in the library.
All objects you want to package into a library must have the same version number. The fpga_libtool command creates libraries encapsulated in operating-system-specific archive files (.a on Linux* and .lib on Windows*). You cannot use libraries created on one operating system with an Intel® high-level design product running on a different operating system.
Create a library file using the following command:
fpga_libtool file1 file2 ... fileN --target (sycl) --create <library_name>
The command parameters are defined as follows:
Parameter |
Description |
---|---|
file1 file2 ... fileN |
You can specify one or more object files to include in the library. |
--target (sycl) |
Target this library for kernels developed. When you mention the sycl option, --target prepares the library for use with the Intel® oneAPI DPC++/C++ Compiler. |
--create <library_name> |
Allows you to specify the name of the library archive file. Specify the file extension of the library file as .a for Linux-platform libraries. |
Example command:
fpga_libtool lib_rtl.o --target sycl --create lib.a
where, the command packages objects created from RTL source code into a SYCL library called lib.a.
Using Static Libraries
You can include static libraries in your compiler command along with your source files, as shown in the following command:
icpx -fsycl -fintelfpga main.cpp lib.a
SYCL_EXTERNAL extern "C" void foo()