Visible to Intel only — GUID: GUID-D70D623D-88DA-4151-AAF0-C106ACA0E95B
Visible to Intel only — GUID: GUID-D70D623D-88DA-4151-AAF0-C106ACA0E95B
Adding Shapes
After drawing the sine curve, Writing a Graphics Program Overview calls drawshapesto put two rectangles and two ellipses on the screen. The fill flag alternates between $GBORDER and $GFILLINTERIOR:
! DRAWSHAPES - Draws two boxes and two ellipses. ! SUBROUTINE drawshapes( ) USE IFQWIN EXTERNAL newx, newy INTEGER(2) dummy, newx, newy ! ! Create a masking (fill) pattern. ! INTEGER(1) diagmask(8), horzmask(8) DATA diagmask / #93, #C9, #64, #B2, #59, #2C, #96, #4B / DATA horzmask / #FF, #00, #7F, #FE, #00, #00, #00, #CC / ! ! Draw the rectangles. ! CALL SETLINESTYLE( INT2(#FFFF )) CALL SETFILLMASK( diagmask ) dummy = RECTANGLE( $GBORDER,newx(INT2(50)),newy(INT2(-325)), & & newx(INT2(200)),newy(INT2(-425))) dummy = RECTANGLE( $GFILLINTERIOR,newx(INT2(550)), & & newy(INT2(-325)),newx(INT2(700)),newy(INT2(-425))) ! ! Draw the ellipses. ! CALL SETFILLMASK( horzmask ) dummy = ELLIPSE( $GBORDER,newx(INT2(50)),newy(INT2(325)), & & newx(INT2(200)),newy(INT2(425))) dummy = ELLIPSE( $GFILLINTERIOR,newx(INT2(550)), & & znewy(INT2(325)),newx(INT2(700)),newy(INT2(425))) END SUBROUTINE
The call to SETLINESTYLE resets the line pattern to a solid line. Omitting this routine causes the first rectangle to appear with a dashed border, because the drawlines subroutine called earlier changed the line style to a dashed line.
ELLIPSE draws an ellipse using parameters similar to those for RECTANGLE. It, too, requires a fill flag and two corners of a bounding rectangle. The following figure shows how an ellipse uses a bounding rectangle:
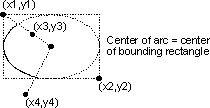
The $GFILLINTERIOR constant fills the shape with the current fill pattern. To create a pattern, pass the address of an 8-byte array to SETFILLMASK. In drawshapes, the diagmaskarray is initialized with the pattern shown in the following table:
Fill Patterns
Bit pattern Value in diagmask Bit No. 7 6 5 4 3 2 1 0 x o o x o o x x diagmask(1) = #93 x x o o x o o x diagmask(2) = #C9 o x x o o x o o diagmask(3) = #64 x o x x o o x o diagmask(4) = #B2 o x o x x o o x diagmask(5) = #59 o o x o x x o o diagmask(6) = #2C x o o x o x x o diagmask(7) = #96 o x o o x o x x diagmask(8) = #4B