Visible to Intel only — GUID: GUID-1EB27F90-5C2B-445D-92E2-974F21B25F6B
Visible to Intel only — GUID: GUID-1EB27F90-5C2B-445D-92E2-974F21B25F6B
Remap
Performs the look-up coordinate mapping of pixels of the source image.
Syntax
Case 1: Operation on pixel-order data
IppStatus ippiRemap_<mod>(const Ipp<datatype>* pSrc, IppiSize srcSize, int srcStep, IppiRect srcRoi, const Ipp32f* pxMap, int xMapStep, const Ipp32f* pyMap, int yMapStep, Ipp<datatype>* pDst, int dstStep, IppiSize dstRoiSize, int interpolation);
Supported values for mod:
8u_C1R |
16u_C1R |
16s_C1R |
32f_C1R |
8u_C3R |
16u_C3R |
16s_C3R |
32f_C3R |
8u_C4R |
16u_C4R |
16s_C4R |
32f_C4R |
16u_AC4R |
16s_AC4R |
32f_AC4R |
IppStatus ippiRemap_<mod>(const Ipp<datatype>* pSrc, IppiSize srcSize, int srcStep, IppiRect srcRoi, const Ipp64f* pxMap, int xMapStep, const Ipp64f* pyMap, int yMapStep, Ipp<datatype>* pDst, int dstStep, IppiSize dstRoiSize, int interpolation);
Supported values for mod:
64f_C1R |
64f_C3R |
64f_C4R |
64f_AC4R |
IppStatus ippiRemap_8u_AC4R(const Ipp<datatype>* pSrc, IppiSize srcSize, int srcStep, IppiRect srcRoi, const Ipp32f* pxMap, int xMapStep, const Ipp32f* pyMap, int yMapStep, Ipp<datatype>* pDst, int dstStep, IppiSize dstRoiSize, int interpolation);
Include Files
ippi.h
Domain Dependencies
Headers: ippcore.h, ippvm.h, ipps.h
Libraries: ippcore.lib, ippvm.lib, ipps.lib
Parameters
pSrc |
Pointer to the source image origin. An array of separate pointers to each plane in case of data in planar format. |
srcSize |
Size, in pixels, of the source image. |
srcStep |
Distance, in bytes, between the starting points of consecutive lines in the source image buffer. |
srcRoi |
Region of interest in the source image (of the IppiRect type). |
pxMap, pyMap |
Pointers to the starts of 2D buffers, containing tables of the x- and y-coordinates. |
xMapStep, yMapStep |
Steps, in bytes, through the buffers containing tables of the x- and y-coordinates. |
pDst |
Pointer to the destination image ROI. An array of separate pointers to ROI in each plane for planar image. |
dstStep |
Distance, in bytes, between the starting points of consecutive lines in the destination image buffer. |
dstRoiSize |
Size of the destination ROI in pixels. |
interpolation |
Specifies the interpolation mode. Possible values are: |
IPPI_INTER_NN - nearest neighbor interpolation |
|
IPPI_INTER_LINEAR - linear interpolation |
|
IPPI_INTER_CUBIC - cubic interpolation |
|
IPPI_INTER_LANCZOS - interpolation with Lanczos window |
|
IPPI_INTER_CUBIC2P_CATMULLROM - Catmull-Rom spline |
|
the following flag is used additionally to the above modes: |
|
IPPI_SMOOTH_EDGE - edge smoothing |
Description
This function operates with ROI (see ROI Processing in Geometric Transforms).
This function transforms the source image by remapping its pixels. Pixel remapping is performed using pxMap and pyMap buffers to look-up the coordinates of the source image pixel that is written to the target destination image pixel. The application has to supply these look-up tables. The remapping of the source pixels to the destination pixels is made according to the following formula:
dst_pixel[i, j]=src_pixel[pxMap[i, j], pyMap[i, j]]
where i,j are the x- and y-coordinates of the target destination image pixel dst_pixel;
pxMap[i, j] contains the x- coordinates of the source image pixels src_pixel that are written to dst_pixel;
pyMap[i, j] contains the y- coordinates of the source image pixels src_pixel that are written to dst_pixel.
If the referenced coordinates correspond to a pixel outside of the source ROI, and the flag IPPI_SMOOTH_EDGE is not set, then no mapping of the source pixel is performed.
Figure “Remapping the Sample Image” gives an example of applying the function ippiRemap to a sample image that is a square grid of alternating blue, red, and green lines.
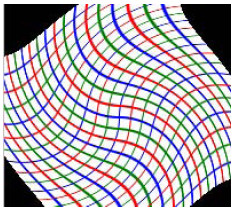
The transformed part of the image is resampled using the interpolation method specified by the interpolation parameter, and is written to the destination image ROI. The function can be used with or without edge smoothing. The pseudo code below shows how it works.
The function works without edge smoothing - the flag IPPI_SMOOTH_EDGE is not set:
if ( xMap < srcRoi . x || xMap > srcRoi . x + srcRoi . width -1 || yMap < srcRoi . y || yMap > srcRoi . y + srcRoi . height -1)
not fill dst /* do not remap */
else
fill dst with Interpolate(Src, xMap, yMap) /* remap */
The function works with edge smoothing - the flag IPPI_SMOOTH_EDGE is set:
if (xMap < srcRoi.x - 1 || xMap > srcRoi.x+srcRoi.width || yMap < srcRoi.y - 1 || yMap > srcRoi.y+srcRoi.height)
not fill dst /* do not remap */
else if (xMap < srcRoi.x || xMap > srcRoi.x+srcRoi.width-1 || yMap < srcRoi.y || yMap > srcRoi.y+srcRoi.height-1)
fill dst with Interpolate(Src, fillvalue, xMap, yMap) /* smoothing */
else
fill dst with Interpolate(Src, xMap, yMap) /* remap */
If the width or height of the input image pSrc is insufficient for the interpolation method specified by the parameter interpolation, the function uses interpolation with a smaller number of pixels for both width and height dimensions. See the following pseudocode:
if (width < 6 || height < 6) { inter== lanczos ? inter = cubic; if ( (width < 4 || height < 4) && ( inter == cubic || inter == catmullrom) ) inter = linear; if (width == 1 || height == 1) inter = nearest; }
Return Values
ippStsNoErr |
Indicates no error. Any other value indicates an error or a warning. |
ippStsNullPtrErr |
Indicates an error condition if one of the specified pointers is NULL. |
ippStsSizeErr |
Indicates an error condition if srcSize or dstRoiSize has a field with zero or negative value. |
ippStsStepErr |
Indicates an error condition if one of the srcStep, dstStep, xMapStep, or yMapStep has a zero or negative value. |
ippStsInterpolationErr |
Indicates an error condition if interpolation has an illegal value. |
ippStsWrongIntersectROIErr |
Indicates an error condition if srcRoi has no intersection with the source image. |
Example
/*******************************************************************************
* Copyright 2015 Intel Corporation.
*
*
* This software and the related documents are Intel copyrighted materials, and your use of them is governed by
* the express license under which they were provided to you ('License'). Unless the License provides otherwise,
* you may not use, modify, copy, publish, distribute, disclose or transmit this software or the related
* documents without Intel's prior written permission.
* This software and the related documents are provided as is, with no express or implied warranties, other than
* those that are expressly stated in the License.
*******************************************************************************/
// A simple example of the source image transformation by remapping
// of its pixels using Intel(R) Integrated Primitives (Intel(R) IPP) function:
// ippiRemap_8u_C1R
#include <stdio.h>
#include "ipp.h"
/* Next two defines are created to simplify code reading and understanding */
#define EXIT_MAIN exitLine: /* Label for Exit */
#define check_sts(st) if((st) != ippStsNoErr) goto exitLine; /* Go to Exit if Intel(R) IPP function returned status different from ippStsNoErr */
int main(void)
{
IppStatus status = ippStsNoErr;
Ipp8u pSrc[4 * 4] =/* Pointers to source images */
{ 0, 1, 2, 3,
4, 5, 6, 7,
8, 9, 0, 1,
2, 3, 4, 5 };
Ipp8u pDst[4*4]; /* Pointers to destination images */
int srcStep = 4, dstStep = 4; /* Steps, in bytes, through the source/destination images */
IppiSize roiSize = { 4, 4 }; /* Size of source/destination ROI in pixels */
Ipp32f pxMap[4 * 4] = {/* Pointer to x map */
0, 1, 2, 3,
0, 1, 2, 0,
0, 1, 0, 0,
0, 1, 2, 3 };
Ipp32f pyMap[4 * 4] = {/* Pointer to y map */
0, 0, 0, 0,
1, 1, 1, 0,
2, 2, 0, 0,
3, 3, 3, 3 };
int xStep = 16, yStep = 16; /* Steps, in bytes, through the x and y maps */
IppiRect rect = { 0, 0, 4, 4 }; /* Region of interest in the source image */
check_sts( status = ippiRemap_8u_C1R(pSrc, roiSize, srcStep, rect, pxMap, xStep, pyMap, yStep,
pDst, dstStep, roiSize, IPPI_INTER_LINEAR) )
EXIT_MAIN
printf("Exit status %d (%s)\n", (int)status, ippGetStatusString(status));
return (int)status;
}