Visible to Intel only — GUID: GUID-51550C92-7C3E-4E59-AB12-17A12CAA5EF0
Visible to Intel only — GUID: GUID-51550C92-7C3E-4E59-AB12-17A12CAA5EF0
FIRGenLowpass
Computes lowpass FIR filter coefficients.
Syntax
IppStatus ippsFIRGenLowpass_64f(Ipp64f rFreq, Ipp64f* pTaps, int tapsLen, IppWinType winType, IppBool doNormal, Ipp8u* pBuffer);
Include Files
ipps.h
Domain Dependencies
Headers: ippcore.h, ippvm.h
Libraries: ippcore.lib, ippvm.lib
Parameters
rFreq |
Normalized cutoff frequency, must be in the range (0, 0.5). |
pTaps |
Pointer to the array where computed tap values are stored. The number of elements in the array is tapsLen. |
tapsLen |
Number of elements in the array containing the tap values; must be equal or greater than 5. |
winType |
Specifies what type of window is used in computations. The winType must have one of the following values:
|
doNormal |
Specifies normalized or non-normalized sequence of the filter coefficients is computed. The doNormal must have one of the following values:
|
pBuffer |
Pointer to the buffer for internal calculations. To get the size of the buffer, use the ippsFIRGenGetBufferSize function. |
Description
This function computes tapsLen coefficients for lowpass FIR filter with the cutoff frequency rFreq by windowing the ideal infinite filter coefficients. The quality of filtering is defined by the number of coefficients. The parameter winType specifies the type of the window. For more information on window types used by the function, see Windowing Functions. The computed coefficients are stored in the array pTaps.
For more information about the used algorithm, see [MIT 93].
Return Values
ippStsNoErr |
Indicates no error. |
ippStsNullPtrErr |
Indicates an error when the pTaps pointer is NULL. |
ippStsSizeErr |
Indicates an error when the tapsLen is less than 5, or rFreq is out of range. |
Example
/*******************************************************************************
* Copyright 2015 Intel Corporation.
*
*
* This software and the related documents are Intel copyrighted materials, and your use of them is governed by
* the express license under which they were provided to you ('License'). Unless the License provides otherwise,
* you may not use, modify, copy, publish, distribute, disclose or transmit this software or the related
* documents without Intel's prior written permission.
* This software and the related documents are provided as is, with no express or implied warranties, other than
* those that are expressly stated in the License.
*******************************************************************************/
#include <stdio.h>
#include "ipp.h"
/* Next two defines are created to simplify code reading and understanding */
#define EXIT_MAIN exitLine: /* Label for Exit */
#define check_sts(st) if((st) != ippStsNoErr) goto exitLine; /* Go to Exit if Intel(R) Integrated Performance Primitives (Intel(R) IPP) function returned status different from ippStsNoErr */
/* Results of ippMalloc() are not validated because Intel(R) IPP functions perform bad arguments check and will return an appropriate status */
int main()
{
int len = 512;
Ipp64f pDst[512];
Ipp64f magn = 4095;
Ipp64f rFreq = 0.2;
int tapslen = 27;
int numIters = 512;
IppsFIRSpec_64f* pSpec = NULL;
IppStatus status = ippStsNoErr;
int i = 0, bufSize = 0, specSize = 0;
Ipp8u* pBuffer = NULL;
IppAlgType algType = ippAlgDirect;
Ipp64f* pDlySrc = NULL;
Ipp64f* pDlyDst = NULL;
Ipp64f* FIRDst = ippsMalloc_64f(numIters * sizeof(Ipp64f));
Ipp64f* taps = ippsMalloc_64f(tapslen*sizeof(Ipp64f));
pDlySrc = ippsMalloc_64f(tapslen*sizeof(Ipp64f));
check_sts( status = ippsZero_64f(pDlySrc, tapslen) )
/*generate source vector*/
check_sts( status = ippsVectorJaehne_64f(pDst, len, magn) ) /* create a Jaehne vector */
printf("\nSource vector\n");
for (i = 0; i < 32; i++) printf("%f,", pDst[i]);
/*computes tapsLen coefficients for lowpass FIR filter*/
check_sts( status = ippsFIRGenGetBufferSize(tapslen, &bufSize) )
pBuffer = ippsMalloc_8u(bufSize);
check_sts( status = ippsFIRGenLowpass_64f(rFreq, taps, tapslen, ippWinBartlett, ippTrue, pBuffer) )
printf("\nGenerated taps:\n");
for (i = 0; i < tapslen; i++) printf("%f,", taps[i]);
check_sts( status = ippsFIRSRGetSize(tapslen, ipp64f, &specSize, &bufSize) )
pSpec = (IppsFIRSpec_64f*)ippsMalloc_8u(specSize);
pBuffer = ippsMalloc_8u(bufSize);
check_sts( status = ippsFIRSRInit_64f(taps, tapslen, algType, pSpec) )
/* filter an input vector */
check_sts( status = ippsFIRSR_64f(pDst, FIRDst, numIters, pSpec, pDlySrc, pDlyDst, pBuffer) )
printf("\nAfter LowPass filter\n");
for (i = 0; i < 32; i++) printf("%f,", FIRDst[i]);
printf("\n");
EXIT_MAIN
ippsFree(FIRDst);
ippsFree(taps);
printf("Exit status %d (%s)\n", (int)status, ippGetStatusString(status));
return (int)status;
}
Result:
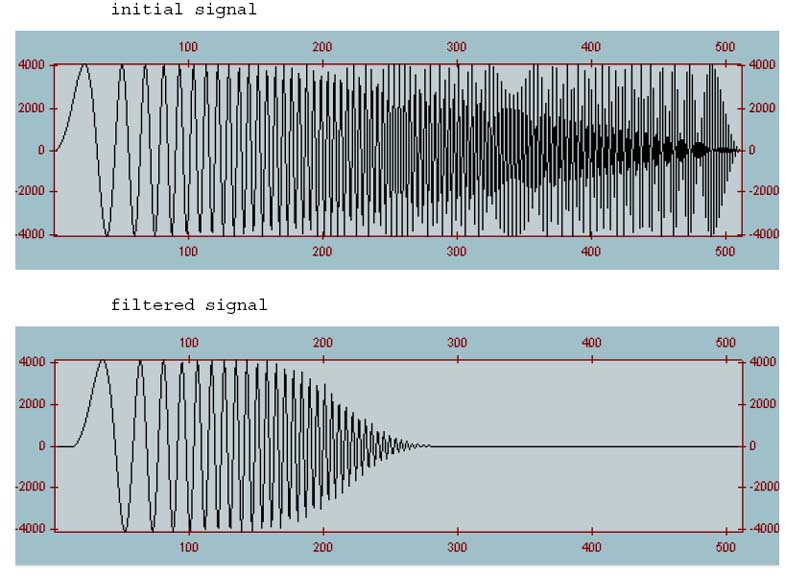