Visible to Intel only — GUID: GUID-1491A97B-7EDE-45BA-8B2C-2F2BB3E4EA3F
Visible to Intel only — GUID: GUID-1491A97B-7EDE-45BA-8B2C-2F2BB3E4EA3F
NLS and MCBS Routines on Windows
The NLS and MCBS routines are only available on Windows* systems. These library routines for handling extended and multibyte character sets are divided into three categories:
Locale Setting and Inquiry routines to set locales (local code sets) and inquire about their current settings
At program startup, the current language and country setting is retrieved from the operating system. The user can change this setting through the Control Panel Regional Settings icon. The current codepage is also retrieved from the system.
There is a system default console codepage and a system default Windows codepage. Console programs retrieve the system console codepage, while Windows programs (including QuickWin applications) retrieve the system Windows codepage.
The NLS Library provides routines to determine the current locale (local code set), to return parameters of the current locale, to provide a list of all the system supported locales, and to set the locale to another language, country and/or codepage. Note that the locales and codepages set with these routines affect only the program or console that calls the routine. They do not change the system defaults or affect other programs or consoles.
NLS Formatting routines to format dates, currency, and numbers
You can set time, date, currency and number formats from the Control Panel, by clicking on the Regional Settings icon. The NLS Library also provides formatting routines for the current locale. These routines return strings in the current codepage, set by default at program start or by NLSSetLocale.
All the formatting routines return the number of bytes in the formatted string (not the number of characters, which can vary if multibyte characters are included). If the output string is longer than the formatted string, the output string is blank padded. If the output string is shorter than the formatted string, an error occurs, NLS$ErrorInsufficientBuffer is returned, and nothing is written to the output string.
Multibyte Character Set (MBCS) routines for using multi-byte characters
Examples of multibyte character sets are Japanese, Korean, and Chinese.
The MBCS inquiry routines provide information on the maximum length of multibyte characters, the length, number and position of multibyte characters in strings, and whether a multibyte character is a leading or trailing byte. The NLS library provides a parameter, MBLenMax, defined in the NLS module to be the longest length (in bytes) of any character, in any codepage. This parameter can be useful in comparisons and tests. To determine the maximum character length of the current codepage, use the MBCurMax function.
There are four MBCS conversion routines. Two convert Japan Industry Standard (JIS) characters to Microsoft Kanji characters or vice versa. Two convert between a codepage multibyte character string and a Unicode string.
There are several MBCS Fortran equivalent routines. They are the exact equivalents of Fortran intrinsic routines except that the MBCS equivalents allow character strings to contain multibyte characters.
Examples
The following example uses Locale Setting and Inquiry routines:
USE IFNLS
INTEGER(4) strlen, status
CHARACTER(40) str
strlen = NLSGetLocaleInfo(NLS$LI_SDAYNAME1, str)
print *, str ! prints Monday
strlen = NLSGetLocaleInfo(NLS$LI_SDAYNAME2, str)
print *, str ! prints Tuesday
strlen = NLSGetLocaleInfo(NLS$LI_SDAYNAME3, str)
print *, str ! prints Wednesday
! Change locale to Spanish, Mexico
status = NLSSetLocale("Spanish", "Mexico")
strlen = NLSGetLocaleInfo(NLS$LI_SDAYNAME1, str)
print *, str ! prints lunes
strlen = NLSGetLocaleInfo(NLS$LI_SDAYNAME2, str)
print *, str ! prints martes
strlen = NLSGetLocaleInfo(NLS$LI_SDAYNAME3, str)
print *, str ! prints miércoles
END
The following example uses NLS Formatting routines:
USE IFNLS
INTEGER(4) strlen, status
CHARACTER(40) str
strlen = NLSFormatTime(str)
print *, str ! prints 11:42:24 AM
strlen = NLSFormatDate(str, flags= NLS$LongDate)
print *, str ! prints Friday, July 14, 2000
status = NLSSetLocale ("Spanish", "Mexico")
strlen = NLSFormatTime(str)
print *, str ! prints 11:42:24
print *, str ! prints viernes 14 de julio de 2000
The following example uses Multibyte Character Set (MBCS) inquiry routines:
USE IFNLS
CHARACTER(4) str
INTEGER status
status = NLSSetLocale ("Japan")
str = " ·, " ¿"
PRINT '(1X,''String by char = '',\)'
DO i = 1, len(str)
PRINT '(A2,\)',str(i:i)
END DO
PRINT '(/,1X,''MBLead = '',\)'
DO i = 1, len(str)
PRINT '(L2,\)',mblead(str(i:i))
END DO
PRINT '(/,1X,''String as whole = '',A,\)',str
PRINT '(/,1X,''MBStrLead = '',\)'
DO i = 1, len(str)
PRINT '(L1,\)',MBStrLead(str,i)
END DO
END
This code produces the following output for str = · , " ¿
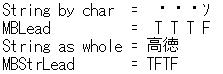
The following example uses Multibyte Character Set (MBCS) Fortran equivalent routines:
USE IFNLS
INTEGER(4) i, len(7), infotype(7)
CHARACTER(10) str(7)
LOGICAL(4) log4
data infotype / NLS$LI_SDAYNAME1, NLS$LI_SDAYNAME2, &
& NLS$LI_SDAYNAME3, NLS$LI_SDAYNAME4, &
& NLS$LI_SDAYNAME5, NLS$LI_SDAYNAME6, &
& NLS$LI_SDAYNAME7 /
WRITE(*,*) 'NLSGetLocaleInfo'
WRITE(*,*) '----------------'
WRITE(*,*) ' '
WRITE(*,*) 'Getting the names of the days of the week...'
DO i = 1, 7
len(i) = NLSGetLocaleInfo(infotype(i), str(i))
WRITE(*, 11) 'len/str/hex = ', len(i), str(i), str(i)
END DO
11 FORMAT (1X, A, I2, 2X, A10, 2X, '[', Z20, ']')
WRITE(*,*) ' '
WRITE(*,*) 'Lexically comparing the names of the days...'
DO i = 1, 6
log4 = MBLGE(str(i), str(i+1), NLS$IgnoreCase)
WRITE(*, 12) 'Is day ', i, ' GT day ', i+1, '? Answer = ', log4
END DO
12 FORMAT (1X, A, I1, A, I1, A, L1)
WRITE(*,*) ' '
WRITE(*,*) 'Done.'
END
This code produces the following output when the locale is Japan:
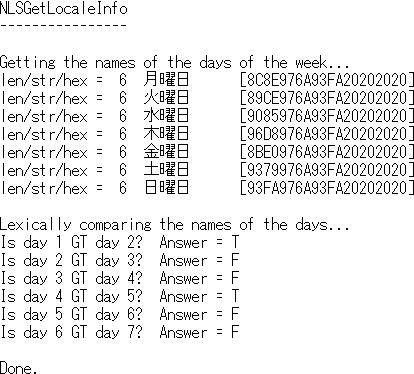